JWT Tokens in Node.js: Best Practices and Common Pitfalls
- Dipen Majithiya
- Jun 17, 2024
- 4 min read
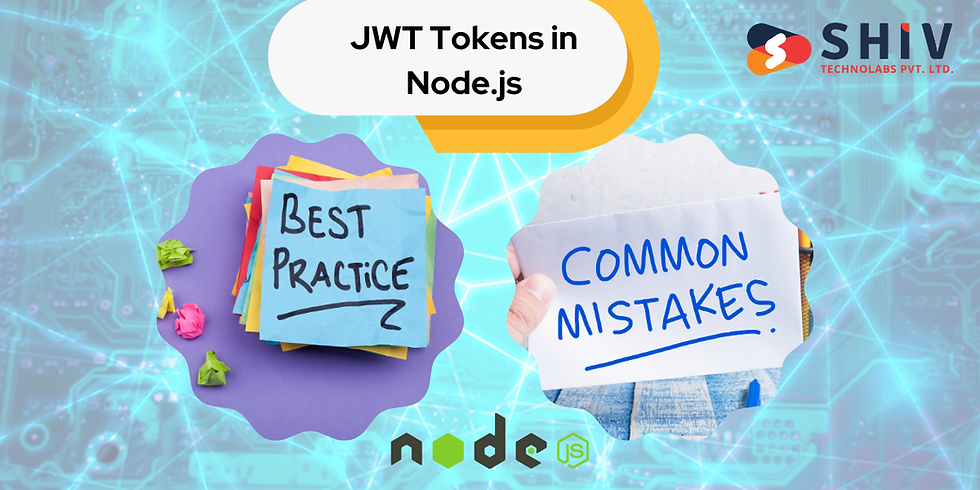
Introduction
JWT tokens have become a standard method for securing APIs and managing authentication in modern web applications. In this blog, we'll discuss the best practices for using JWT tokens in Node.js and highlight common pitfalls to avoid.
Understanding how to implement JWT tokens correctly can significantly improve the security of your application. Let's delve into the details of JWT tokens and their usage in Node.js environments. As a leading Node.js development company in Australia, we are here to guide you through this process.
What are JWT Tokens?
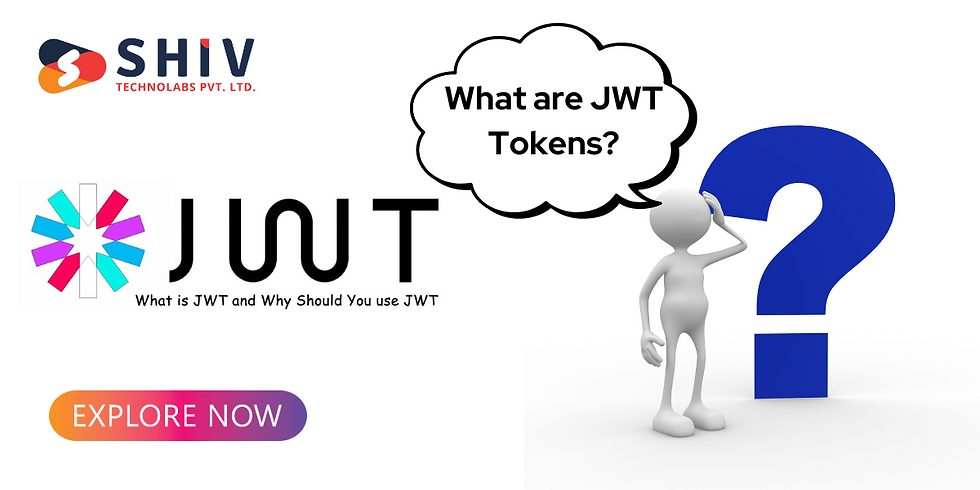
JWT, or JSON Web Tokens, are a compact, URL-safe means of representing claims to be transferred between two parties. They are commonly used for authentication and information exchange.
A JWT consists of three parts: a header, a payload, and a signature. The header typically consists of two parts: the type of token (JWT) and the signing algorithm being used. The payload contains the claims, and the signature ensures that the token hasn’t been tampered with.
Implementing JWT Tokens in Node.js
Setting up JWT tokens in a Node.js application involves several steps. We will go through setting up the environment, installing necessary packages, and writing the code to create and verify tokens.
Setting Up a Node.js Environment
Before you start, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Once installed, create a new project directory and initialize it with npm init.
Also Read: How To Install Node.Js On Windows?
Installing Required Packages
To work with JWT tokens in Node.js, you need the jsonwebtoken package. Install it using the following command:
npm install jsonwebtoken
Creating and Signing a JWT Token
Create a new file, app.js, and write the following code to create and sign a JWT token:
const jwt = require('jsonwebtoken');
const secretKey = 'your_secret_key';
const token = jwt.sign({ username: 'user1' }, secretKey, { expiresIn: '1h' });
console.log('Generated Token:', token);
Verifying a JWT Token
To verify the token, use the verify method provided by the jsonwebtoken package:
jwt.verify(token, secretKey, (err, decoded) => {
if (err) {
console.log('Token verification failed:', err);
} else {
console.log('Decoded Token:', decoded);
}
});
Best Practices for Using JWT Tokens
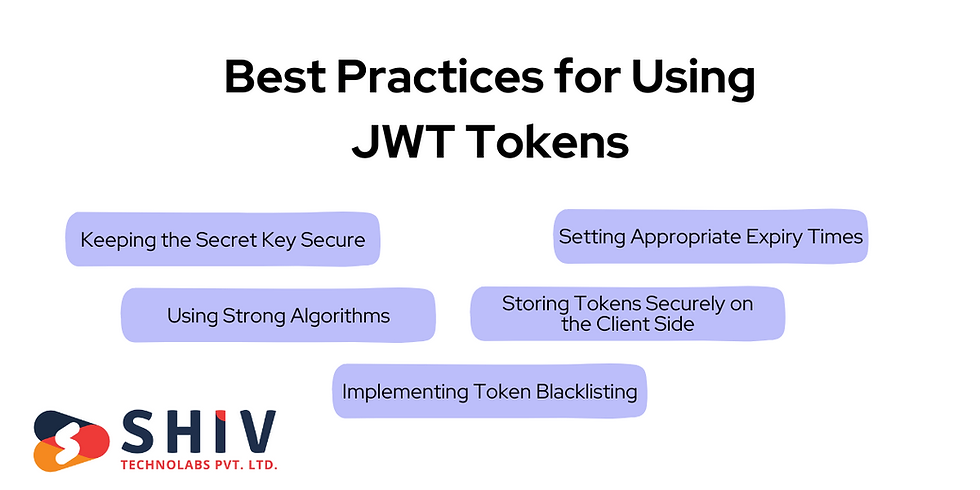
When using JWT tokens, adhering to best practices is crucial for maintaining security and functionality. Here are some key practices to follow.
Keeping the Secret Key Secure
The secret key used to sign JWT tokens must be kept confidential. Exposure of this key can lead to unauthorized access to protected resources. Store it securely in environment variables or configuration management tools.
Setting Appropriate Expiry Times
Always set an expiration time (exp) for your JWT tokens. This limits the window of opportunity for attackers to use stolen tokens. Shorter expiry times enhance security but require more frequent token refreshes.
Using Strong Algorithms
When creating JWT tokens, choose strong algorithms like HS256 or RS256 for signing. Avoid using weaker algorithms, as they are more susceptible to attacks.
Storing Tokens Securely on the Client Side
On the client side, store JWT tokens in secure storage mechanisms like HTTP-only cookies or secure storage APIs. Avoid local storage or session storage for storing sensitive information.
Implementing Token Blacklisting
Token blacklisting allows you to invalidate tokens that should no longer be valid. Implement a mechanism to maintain a blacklist of tokens, checking against this list during token verification.
Common Pitfalls to Avoid
While JWT tokens are powerful, they can introduce security risks if not used correctly. Avoid these common pitfalls to keep your application secure.
Not Validating Tokens Properly
Failing to validate tokens properly can lead to security breaches. Always validate the token's signature and claims, and check for token expiry during each request.
Using Weak Secret Keys
Using weak or predictable secret keys compromises the security of your JWT tokens. Generate strong, random keys and rotate them periodically to maintain security.
Failing to Rotate Keys Regularly
Regular key rotation helps mitigate the risk of key exposure. Implement a process for rotating keys and invalidating tokens signed with old keys.
Storing Sensitive Information in the Payload
Avoid storing sensitive information such as passwords or personal data in the JWT payload. Remember that the payload is base64 encoded, not encrypted, and can be easily decoded.
Ignoring Token Expiry
Ignoring the token's expiry date can lead to long-lived tokens that pose a security risk. Always enforce token expiration and implement a token refresh mechanism to issue new tokens as needed.
Security Considerations
Properly securing JWT tokens involves understanding and mitigating potential vulnerabilities. Regular security audits and careful handling of token revocation are critical steps.
Mitigating JWT Vulnerabilities
To mitigate JWT vulnerabilities, always validate tokens, use strong algorithms, and keep the secret key secure. Regularly review and update your security practices.
Regular Security Audits
Conduct regular security audits to identify and address potential vulnerabilities in your JWT implementation. Staying proactive can help prevent security breaches.
Handling Token Revocation
Implementing token revocation mechanisms is essential for maintaining security. This can involve maintaining a blacklist of invalid tokens or using other techniques to invalidate tokens as needed.
Conclusion
Using JWT tokens in Node.js can significantly improve the authentication process in your applications. By following best practices and avoiding common pitfalls, you can secure your application effectively.
Implementing JWT correctly requires careful attention to detail and ongoing vigilance. Stay updated with the latest security practices and ensure your JWT implementation is secure. For more information and expert help, consider consulting with professionals like Shiv Technolabs. Our skilled Node.js developers in Australia can assist you in building secure and efficient applications.
Comments